How Do You Use a Weather API In Python?
In order to use any API in Python you need to choose an API that provides data in a format that is compatible with Python, like Tomorrow.io does
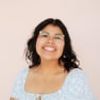
Updated December 25, 2023.
Getting started with Tomorrow.io’s weather API in Python is also pretty simple. In order to use any API in Python you need to choose an API that provides data in a format that is compatible with Python, like Tomorrow.io does. First, you will need to sign up for a free API key to use this service.
Once you have your API key, you can start coding in Python. Here are the steps you can follow:
- Import the required libraries: You will need to import the requests library to make HTTP requests and the json library to handle the JSON response.
import requests
import json
- Set up the API request: Construct a URL with the API endpoint and the necessary parameters, including your API key, the location you want to get the weather for, and the time of the weather forecast you want to retrieve.
You can also specify additional parameters, such as the number of hours or days for which you want to retrieve the forecast data.url = 'https://api.tomorrow.io/v4/timelines'
params = {
'location': 'New York',
'fields': 'temperature',
'units': 'imperial',
'apikey': 'your_api_key',
'timesteps': '1h',
'timezone': 'America/New_York',
'endtime': '2023-03-31T12:00:00Z'
}
Let’s go over the same example we did before, getting temperature data for the state of New York. Along with the forecast we also want hourly timestamp temperatjures ending at 12:00 PM Eastern Time on March 31, 2023.
- Send the API request: Use the requests library to send a GET request to the API endpoint with the parameters you specified. response = requests.get(url, params=params)
- Parse the API response: Use the json library to parse the JSON response into a Python dictionary. data = json.loads(response.text)
- Extract the relevant information: Once you have the response data in a Python dictionary, you can extract the relevant information you want to use in your application. For example, you can get the current temperature in Fahrenheit by accessing the 'value' key in the 'temperature' dictionary. temperature = data['data']['timelines'][0]['intervals'][0]['values']['temperature']
- Display the information: Finally, you can display the weather information in your application. You can print the temperature to the console or use it to update a user interface element in your application. print(f"The current temperature in New York is {temperature} degrees Fahrenheit.")
That's it! With these steps, you can use Python weather API with Tomorrow.io to retrieve and display weather information. Note that there are many other parameters and options you can use with the Tomorrow.io API to customize your requests and retrieve different types of weather data.