How to Fetch Weather API in JavaScript
Display real-time weather data in your app with this easy-to-follow JavaScript guide to integrating a weather API
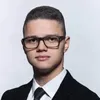
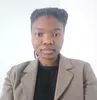
Updated March 5, 2024.
Real-time weather data is now essential for many web applications. Accurate weather information drives decisions in various sectors, from event management to farming. By integrating a weather API, developers can provide their apps with real-time, accurate forecasts.
This article will explore methods for fetching and utilizing weather data in JavaScript. As a flexible language integral to web development, JavaScript provides effective tools for working with weather APIs. We will dive into making API calls from JavaScript, handling the response, and displaying weather information.
Obtaining API Access
First, register an account with Tomorrow.io by signing up and obtaining API access. So, all you have to do is fill out the form below, and the entire registration process will be done within a few minutes.
Once you finish the registration, you can find your API credentials under the API Management section. You'll find your API key here:
Making Requests with a Weather API JavaScript
Now that we've obtained our API key, the next step is to set up the development environment and see how to fetch weather API in JavaScript.
First, you need to create a `.js` file such as `app.js`. Then, open a code editor of your choice and paste the following code into the file:
const options = {method: 'GET', headers: {accept: 'application/json'}};
fetch('https://api.tomorrow.io/v4/weather/realtime?location=miami&apikey=YOUR_API_KEY', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
You can find this code snippet in the Tomorrow.io documentation under the Realtime Weather API by choosing JavaScript as your programming language. Just make sure to replace the `YOUR_API_KEY` placeholder with the API key in your account.
Finally, to run the app, type the following command in the terminal:
node app.js
Displaying Weather Data
In case no errors were caught, your output should look something like the following:
{
data: {
time: '2024-02-07T11:51:00Z',
values: {
cloudBase: null,
cloudCeiling: null,
cloudCover: 1,
dewPoint: 10.13,
freezingRainIntensity: 0,
humidity: 81,
precipitationProbability: 0,
pressureSurfaceLevel: 1014.41,
rainIntensity: 0,
sleetIntensity: 0,
snowIntensity: 0,
temperature: 13.38,
temperatureApparent: 13.38,
uvHealthConcern: 0,
uvIndex: 0,
visibility: 16,
weatherCode: 1000,
windDirection: 303.19,
windGust: 10.38,
windSpeed: 2.88
}
},
location: {
lat: 25.774173736572266,
lon: -80.19361877441406,
name: 'Miami, Miami-Dade County, Florida, United States',
type: 'city'
}
}
As you can see, the output contains valuable weather data for the location we provided in the code snippet (Miami, Florida), including information such as rain intensity, visibility, wind speed, and much more. We can then include any of the provided data fields in the response on your app or website.
Weather API Integration in JavaScript
Using a weather API in JavaScript is relatively simple as long as you follow the weather API documentation and stick to the code provided above. Nevertheless, knowing how to fetch weather API in JavaScript is just the beginning—as you move forward with JavaScript and a robust weather API such as Tomorrow.io, you'll learn how to build and understand complex and meaningful weather applications that leverage the power of real-time weather.