How to Parse JSON Response From a Weather API?
Master JSON parsing for weather APIs. Learn to retrieve, parse, and present accurate weather data, enhancing user experiences.
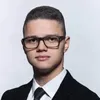
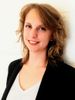
Updated October 24, 2023.
In modern weather applications, detailed weather insights are a must for a dynamic and interactive user experience. Weather APIs offer a wealth of data, encompassing everything from temperatures and historical trends to detailed forecasts and patterns. However, this data usually comes in a JSON format, requiring correct parsing. In this article, we'll cover the essentials when it comes to parsing JSON responses from weather APIs, including retrieving data, understanding JSON structures, and extracting details. Let's get started.
Making API Requests - Example
Let's take a brief look at how to make API requests with JavaScript. To retrieve weather data with JavaScript, use the fetch function as shown in the example below. The code snippet demonstrates how to query the Tomorrow.io API for real-time weather information in Toronto. Of course, remember to replace 'YOUR_API_KEY' with your actual API key in the URL for the code to function correctly. Once the data is received, it's logged to the console. In the event of errors, the .catch() block helps identify and handle any issues during the request process.
const options = {method: 'GET', headers: {accept: 'application/json'}};
fetch('https://api.tomorrow.io/v4/weather/realtime?location=toronto&apikey=YOUR_API_KEY', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
Understanding and Parsing the JSON Response
Once you've fetched weather data using an API request, the next step is understanding and parsing the JSON response. JSON responses from weather APIs are structured hierarchically, featuring nested objects and arrays that hold diverse information. To effectively extract specific details, you need to navigate this structure using keys. For instance, if you're interested in the temperature, you'd access the temperature value within the JSON hierarchy. With programming languages such as JavaScript, functions such as JSON.parse() prove invaluable for converting the raw JSON data into a readable format. Here's how the API request's response looks:
{
"data": {
"time": "2023-08-16T14:16:00Z",
"values": {
"cloudBase": 0.7,
"cloudCeiling": null,
"cloudCover": 34,
"dewPoint": 17.13,
"freezingRainIntensity": 0,
"humidity": 71,
"precipitationProbability": 0,
"pressureSurfaceLevel": 1003.82,
"rainIntensity": 0,
"sleetIntensity": 0,
"snowIntensity": 0,
"temperature": 22.63,
"temperatureApparent": 22.63,
"uvHealthConcern": 1,
"uvIndex": 3,
"visibility": 13.2,
"weatherCode": 1100,
"windDirection": 335.88,
"windGust": 5.13,
"windSpeed": 5.13
}
},
"location": {
"lat": 43.653480529785156,
"lon": -79.3839340209961,
"name": "Toronto, Golden Horseshoe, Ontario, Canada",
"type": "administrative"
}
}
Inside the "data" object key, you'll find multiple weather statistics, including clouds, humidity, temperature, wind speed, and more. Of course, once you have the response, you can pull out any one of these object keys and include them in your app. For instance, here's how you can print the cloudBase value in Toronto using JavaScript.
const options = { method: 'GET', headers: { accept: 'application/json' } };
fetch('https://api.tomorrow.io/v4/weather/realtime?location=toronto&apikey=YOUR_API_KEY', options)
.then(response => response.json())
.then(response => {
const cloudBase = response.data.values.cloudBase;
console.log('Cloud Base:', cloudBase);
})
.catch(err => console.error(err));
Easily Master JSON Parsing
By understanding JSON's structure and using parsing techniques, you can easily extract key details like temperature, humidity, wind speed, and more. With this knowledge, you can create a better user experience by presenting accurate weather insights in an approachable and user-friendly way. Of course, as you explore weather APIs further, your ability to work with JSON responses will help create engaging apps that keep users up-to-date with the latest weather trends.